One of the first things web developers have to figure out is whether or not they intend to work with JavaScript or with TypeScript. Each of these coding languages comes with its own share of advantages as well as drawbacks which can affect the overall structure, maintenance, and cost of your project greatly. In this article, we will cover the particulars of TypeScript vs JavaScript, including its benefits and potential uses. Whether you are professional or a beginner, knowing about the JavaScript vs Typescript controversy will enable you to choose the right side and make the best decision for yourself and your project.
What is TypeScript vs JavaScript?
Before getting to the specifics, it is important to understand the difference between TypeScript vs JavaScript. To put it simply, JavaScript is a web programming language that is object-oriented and can be interpreted to add interactivity to web pages. It is used widely today and is the base of many frameworks and libraries like React, Angular, and Vue.js. In JavaScript, the types are set dynamically, which means they are assigned during program execution rather than in advance at compilation time.
On the contrary, TypeScript is a derivative of JavaScript, adding on optional static typing bridging dynamic and static languages. By using TypeScript, a Developer can specify certain types of variables during development. A TypeScript program will always compile to plain JavaScript for execution in the browser or on a server regardless of its JavaScript base. The key distinction in this TypeScript vs Javascript debate is that TypeScript necessitates the compilation step.
What is the Difference Between TypeScript and JavaScript?
The type system is where TypeScript vs JavaScript differs the most. As mentioned, TypeScript is Statically typed. This implies that the defined types of variables are checked at compilation time and, hence, is associated with relatively fewer bugs. On the other hand, JavaScript is Dynamically typed where variable type errors are checked at runtime. This, in turn, has significant impact on development with these languages.
Differences extend to how both languages treat errors as well. A developer using JavaScript will more commonly run into runtime errors that are challenging to pinpoint until code execution. TypeScript’s static typing on the other hand does eliminate most of these problems if the code is carefully structured, allowing developers to catch many of these errors at compile-time instead.
Key Differences Between TypeScript and JavaScript
In addition to their typing systems, there are several key distinctions between JavaScript vs TypeScript:
1. Error Handling and Debugging
An advantage of TypeScript is that it is able to catch errors within the development process. The static typing of TypeScripts means that preemptive debugging is significantly more manageable because a wide range of problems are flagged prior to the code running. This feature is especially helpful in larger applications when issues during runtime are extremely difficult to track down.
JavaScript developers face the problem of executing code only to find bugs and errors that were not present during the development phase. It becomes extremely cumbersome when trying to debug and test the projects that are scaled up.
2. Autocompletion and IDE Support
The manual type definitions provided by TypeScript boosts the ability of IDEs to Autocomplete and Intellisense. Support for TypeScript in most modern IDEs which provide Not just quicker development but with proper type information, IDEs can give function, method and variable suggestions. Making typo or logic errors far less likely.
JavaScript does not support autocompletion or type inference as dynamically as other programming languages do. A few modern Integrated Development Environments (IDEs) do provide limited assistance for JavaScript, but it certainly cannot compare to what TypeScript offers.
3. Learning Curve and Complexity
If JavaScript is someone’s first programming language, they will find it beginner friendly. With JavaScript, one can start writing code with no concern regarding type annotation or any other advanced concepts. Its flexibility and ease of use makes it a great first language for learners.
TypeScript, on the other hand, can be considered more difficult because of its rigid type system. Developers are expected to be familiar with type annotation, interfaces, and classes. This system adds a new layer of complexity which translates into a longer learning curve, especially for those who are used to JavaScript’s lenient and versatile nature.
That said, many developers consider TypeScript worth the investment because it greatly decreases the amount of bugs and increases scalability. And though TypeScript is rather difficult for new learners and requires more effort, it becomes increasingly valuable when working on large projects as part of a team.
4. Development Speed
The absence of a type system in JavaScript typically enables more rapid web development in the short term. Programmers can easily write and run their code without being concerned about types or the compilation process. That is the reason why JavaScript is frequently selected for small projects, prompt prototypes, or private scripts.
Nevertheless, in more intricate projects, TypeScript can actually improve development speed since it reduces type-related blunders early on. This makes the code more predictable, lessens the time allocated for debugging. It is especially useful in large initiatives, or when working collaboratively, as consistency and maintainability are crucial aspects.
5. Compilation vs. Interpretation
The most significant distinctions between TypeScript vs JavaScript stem from the fact that TypeScript is a compiled language while JavaScript is an interpreted language.
TypeScript code must be transpiled into JavaScript before it can be executed in the browser or on a server. This means that TypeScript developers need to set up a build process or use tools like Webpack or TSC (TypeScript Compiler) to compile the code.
JavaScript, on the other hand, is interpreted directly by the browser or Node.js runtime. This allows for immediate execution of code without any compilation steps, which contributes to its popularity in quick web development cycles.
6. Static vs Dynamic Typing
As mentioned earlier, the main difference between JavaScript and TypeScript is a difference in their typing systems. JavaScript features dynamic typing, which means that a variable is able to assume any value of any type. While this is convenient, it poses a risk during runtime whereby a variable is assigned an incompatible value.
On the other hand, TypeScript is statically typed. This means that unlike JavaScript, where types can change during execution, TypeScript allows for types to be determined during the development stage allowing for more stringent checking during compilation. A good example is if a variable is declared to be a number, it cannot be set to a string type later without causing a compilation error.
Static typing in TypeScript alongside its benefits comes with more reliable outcomes, specifically in huge codes, where types are often missed and reported manually, making the process painful.
7. Tooling and Ecosystem
Due to the more popular use of JavaScript, it has a richer ecosystem with libraries, frameworks, and tools built in support of it. However, TypeScript has become more used over these recent years and so modern popular frameworks such as Angular and React as well as Vue.js support TypeScript natively.
In addition, the TypeScript space is expanding, and several libraries have started incorporating type definitions which help with the management of external dependencies. For instance, TypeScript provides type definitions for numerous npm packages freeing developers from writing excessive boilerplate code and enhancing the overall experience for developers.
8. Community Support
Concerned for nothing, as the ecosystem of both JavaScript and TypeScript is massive, active, and supportive. Given its age, JavaScript hosts many more developers and has resources in the form of tutorials, documentation, blogs, etc. The TypeScript community, although smaller, is seeing growth as more and more developers engage with discussion forums and write tutorials and articles about best practices.
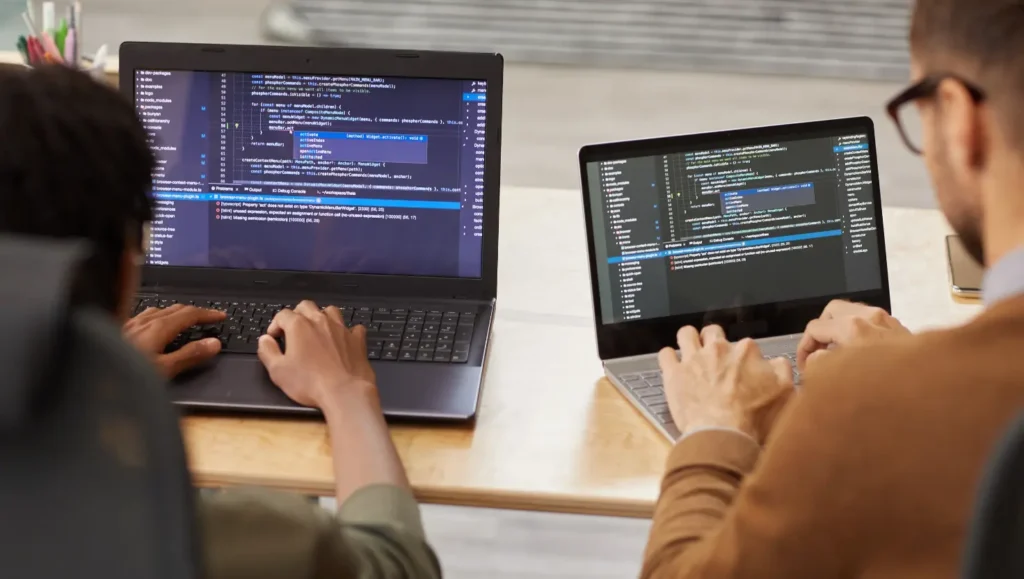
TypeScript vs JavaScript: Key Syntax Differences Explained
As JavaScript is the core foundation of TypeScript, both languages share a comparable structure. Still, TypeScript adds an extra capability in the form of type annotations, interfaces, enums, and even more complex type features. In essence, TypeScript boasts functionalities that JavaScript cannot offer. Some of the major contrasts in the syntax of TypeScript vs JavaScript as mentioned underneath differ from one another.
1. Type Annotations
In TypeScript, you can explicitly specify the type of variables, function parameters, and return values. JavaScript does not have this feature.
Example:
JavaScript:
let message = “Hello, world!”;
TypeScript:
let message: string = “Hello, world!”;
2. Interfaces
TypeScript allows you to define interfaces to specify the shape of an object. JavaScript does not have interfaces.
Example:
TypeScript:
interface User {
name: string;
age: number;
}
const user: User = {
name: “Alice”,
age: 25,
};
JavaScript:
You would achieve this with plain objects or dynamic property handling, but without strict typing:
const user = {
name: “Alice”,
age: 25,
};
3. Classes with Modifiers
TypeScript adds access modifiers like public, private, and protected to class properties and methods.
Example:
TypeScript:
typescript
CopyEdit
class Person {
private name: string;
constructor(name: string) {
this.name = name;
}
getName(): string {
return this.name;
}
}
JavaScript:
In JavaScript, access modifiers are not available. Private fields are denoted with #, but this is a more recent addition and less flexible.
javascript
CopyEdit
class Person {
#name;
constructor(name) {
this.#name = name;
}
getName() {
return this.#name;
}
}
Summary Table:
Feature | JavaScript Syntax | TypeScript Syntax |
Static Typing | N/A | let x: number = 42; |
Interfaces | Not supported | interface User { name: string; age: number; } |
Enums | Simulated via objects | enum Color { Red, Green, Blue } |
Generics | Not supported | function identity<T>(arg: T): T { return arg; } |
Classes with Modifiers | Public by default | private, protected, and public modifiers |
Tuples | Regular arrays | [string, number] |
Optional Parameters | Handled dynamically | function greet(name: string, age?: number) |
Type Assertions | Type coercion | (value as string) or <string>value |
By using TypeScript’s additional features, developers gain more control over their code and reduce errors, especially in large or complex applications.
When Should You Use TypeScript vs JavaScript?
Now that we’ve explored the key differences between TypeScript vs JavaScript, let’s talk about when you should choose one over the other.
Use TypeScript if:
- You’re working on a large-scale project: In extensive codebases, TypeScript is extremely useful as it helps with type safety, maintainability, and error detection.
- You’re working with a team: TypeScript’s static typing makes it easier for team members to understand each other’s code, leading to better collaboration and fewer bugs.
- You need better tooling and IDE support: Features such as autocompletion, type inference, and type definitions make Development with modern tools and frameworks significantly smoother in TypeScript.
- You want to reduce runtime errors: If you value catching errors at compile time, you will find TypeScript’s static typing can safeguard you against a multitude of bugs.
Use JavaScript if:
- You’re working on small projects or prototypes: Javascript offers great flexibility and enables fast development which is great for small projects and prototypes.
- You want a simple, straightforward language: JavaScript becomes the solution if you wish to avoid additional complexity that comes with type annotations and compilation.
- You’re familiar with JavaScript and have no time to learn TypeScript: If you have a working knowledge of JavaScript already and don’t require TypeScript, it probably isn’t worth the hassle of switching.
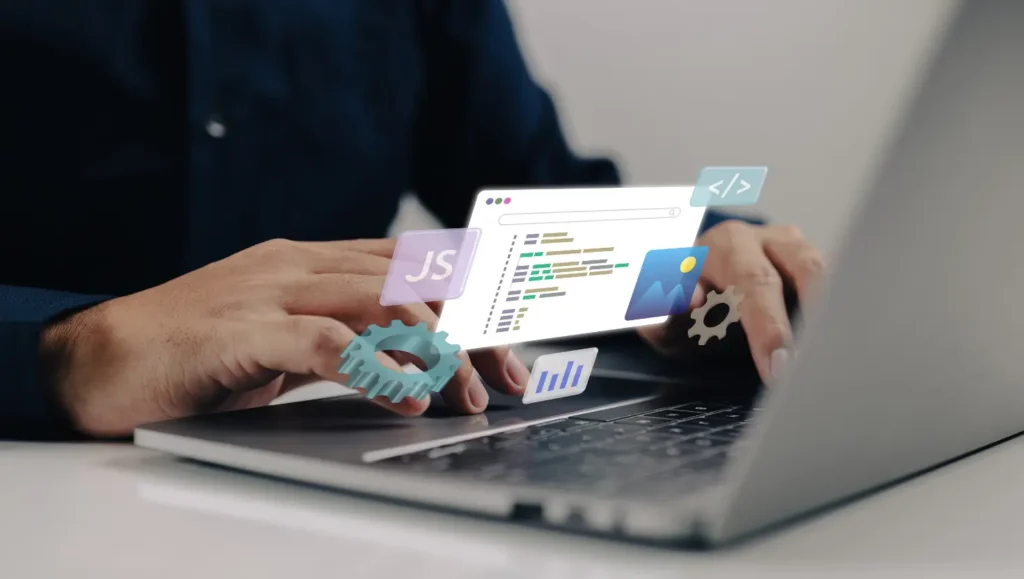
Conclusion: TypeScript vs JavaScript
The crux of the TypeScript vs JavaScript debate comes down to the size of the project, its complexity, and the developer’s choice. TypeScript is flexible and easy to use, allowing for quick development cycles and being perfect for smaller projects. However, for bigger projects with complex data structures that require long-term maintenance, TypeScript provides a more robust framework.
Familiarity with the fundamental differences of TypeScript vs JavaScript enables informed decisions on the ideal programming language to utilize based on available resources, team size, and the type of application they intend to develop. Each of these programming languages has its pros and cons, but when used prudently, TypeScript enables a more orderly and less problematic development process than JavaScript, while JavaScript still remains the desired option for quickness and ease on smaller projects.
Faqs:
1. Which runs faster: TypeScript vs JavaScript?
JavaScript is usually faster as it performs better in terms of runtime. This is due to JavaScript not needing the compilation step that TypeScript does. However, TypeScript improves the overall Web development speed by giving better tooling support and encouraging fewer bugs in the system. This ultimately leads to a faster development cycle and less errors in JavaScript.
2. Is JavaScript better than TypeScript?
The TypeScript vs JavaScript argument is not a straightforward matter. Those in favor of TypeScript argue that it works best for very large applications while those in favor of JavaScript argue that its simplicity is beneficial for simple projects, speedy development, and even prototypes.
3. Can JavaScript libraries be used in TypeScript?
Yes, TypeScript integrates fully with JavaScript libraries. TypeScript works out of the box with a lot of popular JavaScript libraries because they have type definitions. For libraries that do not come with type definitions, developers can always find third party add-ons or write their own type definitions.
4. When is JavaScript the more appropriate option to utilize?
The ever-present bone of contention TypeScript vs JavaScript leans in favor of JavaScript for small implementations, prototyping or personal scripts where there is no compelling reason to add the complexity that comes with TypeScript. In addition, when the end goal is achieved quickly, JavaScript surely wins.
5. Why should I choose TypeScript over JavaScript?
When it comes to TypeScript vs JavaScript, TypeScript offers a more secure and productive development environment in terms of error checking, unit testing, and team collaboration, making it the best choice for extensive and intricate projects
6. Is JavaScript better than TypeScript?
The TypeScript vs JavaScript argument is not a straightforward matter. Those in favor of TypeScript argue that it works best for very large applications while those in favor of JavaScript argue that its simplicity is beneficial for simple projects, speedy development, and even prototypes